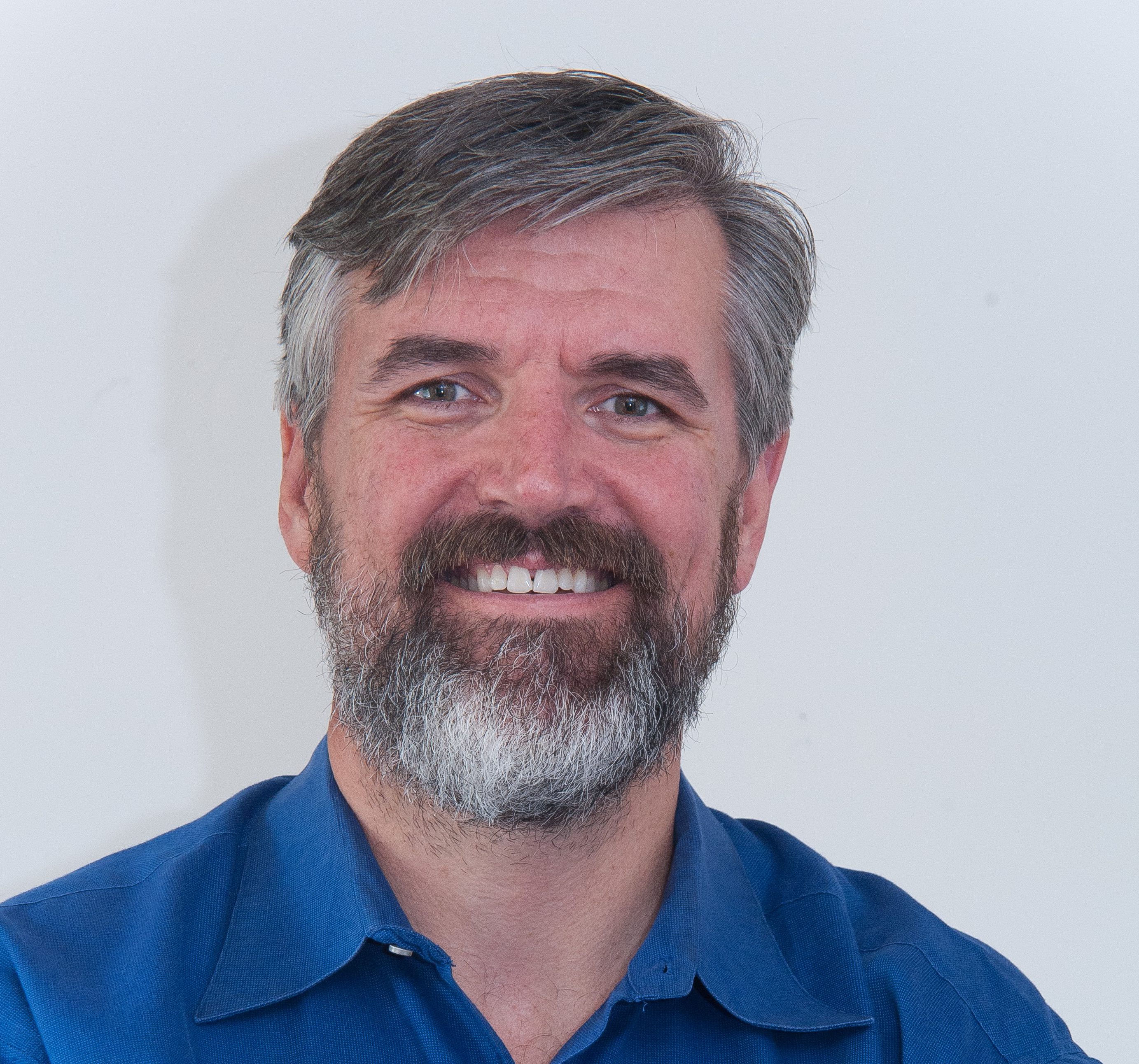
Amazon Lambda – E-mail via SNS the URL of an S3 file when created
I threw together the following Lambda function to send an e-mail via SNS (Amazon’s Simple Notification Service) whenever a file is created in S3 (Amazon’s Simple Storage Service.) Yes, it’s barely commented and overly loggy, but you get the idea:
'use strict'; console.log('Loading function'); const aws = require('aws-sdk'); const s3 = new aws.S3({ apiVersion: '2006-03-01' }); exports.handler = (event, context, callback) => { //console.log('Received event:', JSON.stringify(event, null, 2)); // Get the object from the event and show its content type const bucket = event.Records[0].s3.bucket.name; const key = decodeURIComponent(event.Records[0].s3.object.key.replace(/\+/g, ' ')); const params = { Bucket: bucket, Key: key, }; s3.getObject(params, (err, data) => { if (err) { console.log(err); const message = `Error getting object ${key} from bucket ${bucket}. Make sure they exist and your bucket is in the same region as this function.`; console.log(message); callback(message); } else { //console.log(err); const URLMessage = `https://s3-us-west-2.amazonaws.com/supportacdcalls/${key} `; const SuccessMessage = `Success getting object ${key} from bucket ${bucket}. `; console.log(SuccessMessage); console.log(URLMessage); //console.log('CONTENT TYPE:', data.ContentType); //Send SNS var sns = new aws.SNS(); sns.publish({ TopicArn: 'arn:aws:sns:us-west-2:xxxxxxxxxxxx:RecordingNotificationEmail', Message: JSON.stringify(URLMessage) }, function(err, data) { if(err) { console.error('error publishing to SNS'); context.fail(err); } else { console.log('message published to SNS'); context.done(null, data); } }); callback(null, data.ContentType); } }); };
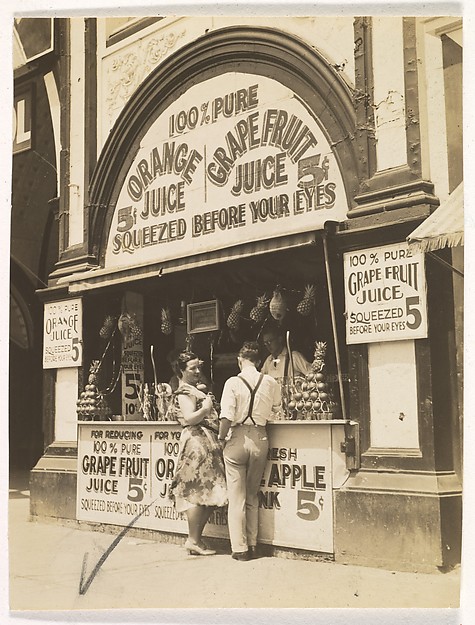
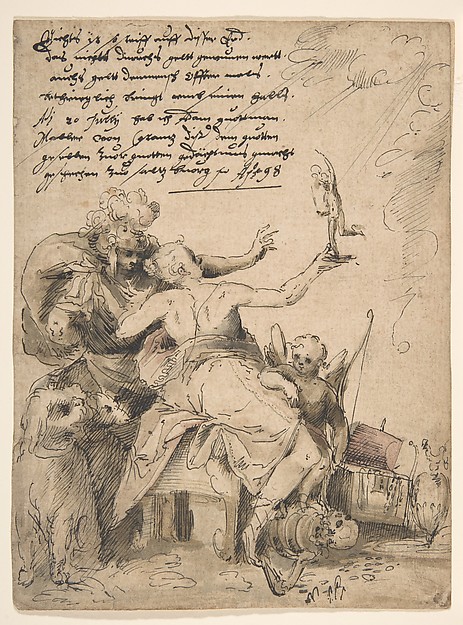
2 Comments
Roy
Thanks for this. I created an event on my S3 bucket that calls on this lambda function. I copy/pasted the lambda function you created above into a new function (node.js 8.10) and made changes to the aws region where bucket is located and the arn information.
When I test it, I get the following error: TypeError: Cannot read property ‘0’ of undefined
at exports.handler (/var/task/index.js:14:30)
When uploading new objects to the bucket, it does not appear to trigger the lambda function either even though I created the event on the bucket to send to this lambda function.
Any ideas what I may have done wrong?
Thanks,
Roy
Mike
Hi Roy – That’s a good question. The guess off the top of my head would be AWS security, that the Lambda script doesn’t have the correct permissions. See if that points you in the right direction.